Getting started with currencystack
Thanks for trying out currencystack! Here, we will give you a walk-through on how to get your app or website set up with currencystack's curency conversion API. The code snippets below will show elementary integration with Ruby, PHP, and Node, but as every API, this is both language and framework agnostic: you get vanilla JSON, so any programming environment with a JSON library can use currencystack.io
We assume you have currencystack account and thereby have an API key to make requests. Please, change the apikey to your own.
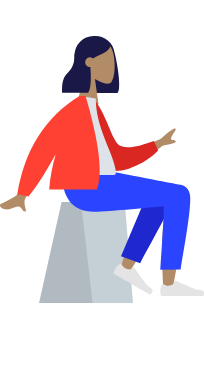
Making your first request
Making a request for geolocation data with currencystack is pretty simple. Try running this in the command line:
curl -X GET \
'https://api.currencystack.io/currency?base=USD&target=EUR,GBP&apikey=API_KEY'
That should return a JSON object that maybe looks something like this:
{
"base": "USD",
"last_update": "2018-12-10T15:10:58.253Z",
"rates": {
"EUR": 0.8769574627,
"GBP": 0.7914459201
},
"status": 200,
"target": "EUR,GBP"
}
Clear and simple.
cURL
curl -X GET \
'https://api.currencystack.io/currency?base=USD&target=EUR,GBP&apikey=API_KEY'
PHP

With HttpRequest
$request = new HttpRequest();
$request->setUrl('https://api.currencystack.io/currency?base=USD&target=EUR,GBP');
$request->setMethod(HTTP_METH_GET);
$request->setQueryData(array(
'apikey' => 'API_KEY'
));
try {
$response = $request->send();
echo $response->getBody();
} catch (HttpException $ex) {
echo $ex;
}
With cURL
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.currencystack.io/currency?base=USD&target=EUR,GBP&apikey=API_KEY",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"cache-control: no-cache",
"postman-token: 8c102565-006a-9496-c414-65a7b7a00e20"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
Python
Python http.client (Python3)
import http.client
conn = http.client.HTTPSConnection("api.currencystack.io")
headers = {
'cache-control': "no-cache",
'postman-token': "af79289d-56ce-8e00-16e2-43a279b931e0"
}
conn.request("GET", "/currency?base=USD&target=EUR,GBP&apikey=API_KEY",
headers=headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
With Request
import requests
url = "https://api.currencystack.io/ip/197.164.124.28/json"
querystring = {"apikey":"API_KEY"}
headers = {
'cache-control': "no-cache",
'postman-token': "95b5b551-7870-b00f-b554-d4f41631bf3b"
}
response = requests.request("GET", url, headers=headers, params=querystring)
print(response.text)
Nodejs
native
var http = require("https");
var options = {
"method": "GET",
"hostname": "api.currencystack.io",
"port": null,
"path": "/currency?base=USD&target=EUR,GBP&apikey=API_KEY"
};
var req = http.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function () {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
});
req.end();
With Request
var request = require("request");
var options = { method: 'GET',
url: 'https://api.currencystack.io/ip/197.164.124.28/json',
qs: { apikey: 'API_KEY' }};
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});
Ruby
With NET:Http
require 'uri'
require 'net/http'
url = URI("https://api.currencystack.io/currency?base=USD&target=EUR,GBP&apikey=API_KEY")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
response = http.request(request)
puts response.read_body
Go
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.currencystack.io/currency?base=USD&target=EUR,GBP&apikey=API_KEY"
req, _ := http.NewRequest("GET", url, nil)
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := ioutil.ReadAll(res.Body)
fmt.Println(res)
fmt.Println(string(body))
}
Java
OK HTTP
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://api.currencystack.io/currency?base=USD&target=EUR,GBP&apikey=API_KEY")
.get()
.build();
Response response = client.newCall(request).execute();
Unirest
HttpResponse response = Unirest.get("https://api.currencystack.io/currency?base=USD&target=EUR,GBP&apikey=API_KEY")
.asString();
Swift
NSURL
import Foundation
let request = NSMutableURLRequest(url: NSURL(string: "https://api.currencystack.io/currency?base=USD&target=EUR,GBP&apikey=API_KEY")! as URL,
cachePolicy: .useProtocolCachePolicy,
timeoutInterval: 10.0)
request.httpMethod = "GET"
let session = URLSession.shared
let dataTask = session.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
if (error != nil) {
print(error)
} else {
let httpResponse = response as? HTTPURLResponse
print(httpResponse)
}
})
dataTask.resume()